MAFUITableView and MAFUITableViewCell extend the native UITableView and
UITableViewCell classes with MAF styling capability using the MAFStyling
protocol.
You can populate the MAFUITableView with MAFUITableViewCells. Cells are grouped by sections:
MAFUITableView and MAFUITableViewCell – Plain and Grouped Table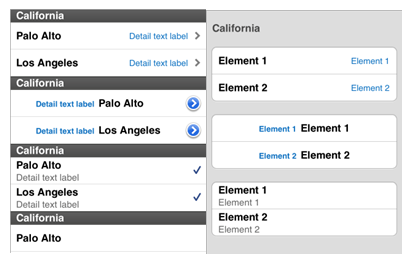
Construct and populate the table view in the same way as for the native table
view:
/*The interface of the ViewController implementing the MAFUITableView*/
#import <UIKit/UIKit.h>
#import "MAFUITableView.h"
@interface ViewController1 : UIViewController<UITableViewDelegate, UITableViewDataSource> {
NSMutableArray* m_TableData;
MAFUITableView* m_TableView;
}
@property (nonatomic, retain) NSMutableArray* tableData;
@property (nonatomic, retain) MAFUITableView* tableView;
@end
The UIViewController must implement the two UITableView delegates. m_TableView is a
reference to the MAFUITableView instance. NSMutableArray is the example data store
for the table view. This is one of the many ways provided by the iOS platform to
construct a table view interface. The implementation of the controller is similar to
constructing a native
UITableView:
/*Implement required methods from UITableViewDataSource Protocol*/
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section;
// Row display. Implementers should *always* try to reuse cells by setting each cell's reuseIdentifier and querying for available reusable cells with dequeueReusableCellWithIdentifier:
// Cell gets various attributes set automatically based on table (separators) and data source (accessory views, editing controls)
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
…
MAFUITableViewCell *cell = [self.tableView dequeueReusableCellWithIdentifier:cellIdentifier];
if (cell == nil) {
cell = [[[MAFUITableViewCell alloc] initWithStyle: UITableViewCellStyleValue1
reuseIdentifier: cellIdentifier] autorelease];
}
…
}
To present a grouped MAFUITableView, use the appropriate constructor call and pass
the style parameter to
it:
MAFUITableView* tempTableView = [[MAFUITableView alloc] initWithFrame:CGRectMake(10, 10, 320, 400) style:UITableViewStyleGrouped];
An
alternative way to do this is to use the Storyboard UI designer, which is built into
Xcode.
To change the appearance of the MAFUITableView and the MAFUITableViewCell classes,
declare your custom style XML classes:
<!-- ListBox is mapped to TableView on iOS -->
<Style basedOn="ListBox" Key="myListBox" TargetType="ListBox" platform="ios">
<Setter Property="Background" Value="#BBCCFF"/>
<Border BorderBrush="#FF9595" BorderThickness="4" CornerRadius="8" />
</Style>
<!-- ListBoxItem is mapped to TableViewCell on iOS -->
<Style BasedOn="ListBoxItem" Key="myListBoxItem"
TargetType="ListBoxItem" platform="ios">
<Setter Property="DetailsFontFamily" Value="Helvetica-Bold"/>
<Setter Property="DetailsFontSize" Value="17"/>
<Setter Property="DetailsTextColor" Value="#666666"/>
</Style>
To apply your custom style classes, set the key value to match the mafStyleName
properties of the
controls:
/* set custom style for table view */
…
tempTableView.mafStyleName = @"myListbox";
/* set custom style for table view cells*/
…
cell.mafStyleName = @"myListboxItem";
You can change these properties of the MAFUITableView in the skinning XML:
Background |
Color of the background of the MAFUITableView; can only be
a solid color, no freestyle gradient. Colors are defined as RGBA
(red, green, blue, alpha). |
SeparatorColor |
The MAFUITableView's cell separator color. |
Border |
The border attributes of the MAFUITableView include: - Borderbrush – defines the color.
- BorderThickness – is the width of the border.
- CornerRadius – is the angle of the edge.
|
You can change these properties of the MAFUITableViewCell in the skinning XML:
Background |
Color for the TableViewCell background, it can be a solid
color, freestyle gradient, ios style background color, colors
are difined as RGBA (red, green, blue, alpha). |
Foreground |
The tableviewcell's textcolor. |
FontFamily |
The tableviewcell's fontfamily. |
FontSize |
The tableviewcell's fontsize. Every tableviewcell can have 2
textsLabels, the secondary is called detailsTextlabel. It can
alsobe styled . |
DetailsForeground |
The tableviewcell's detailtextcolor (the textcolor of the
secondary text). |
DetailsFontFamily |
The tableviewcell's detailfontfamily. |
DetailsFontSize |
The tableviewcell's detailfontsize. |
HorizontalContentAlignment |
The horizontal alignment for the tableviewcell's text.
|
VerticalContentAlignment |
The vertical alignment for the tableviewcell's text.
|
Border |
The border attributes of the MAFUILabel include: - Borderbrush – defines the color.
- BorderThickness – is the width of the border.
- CornerRadius – is the angle of the edge.
|